Blog
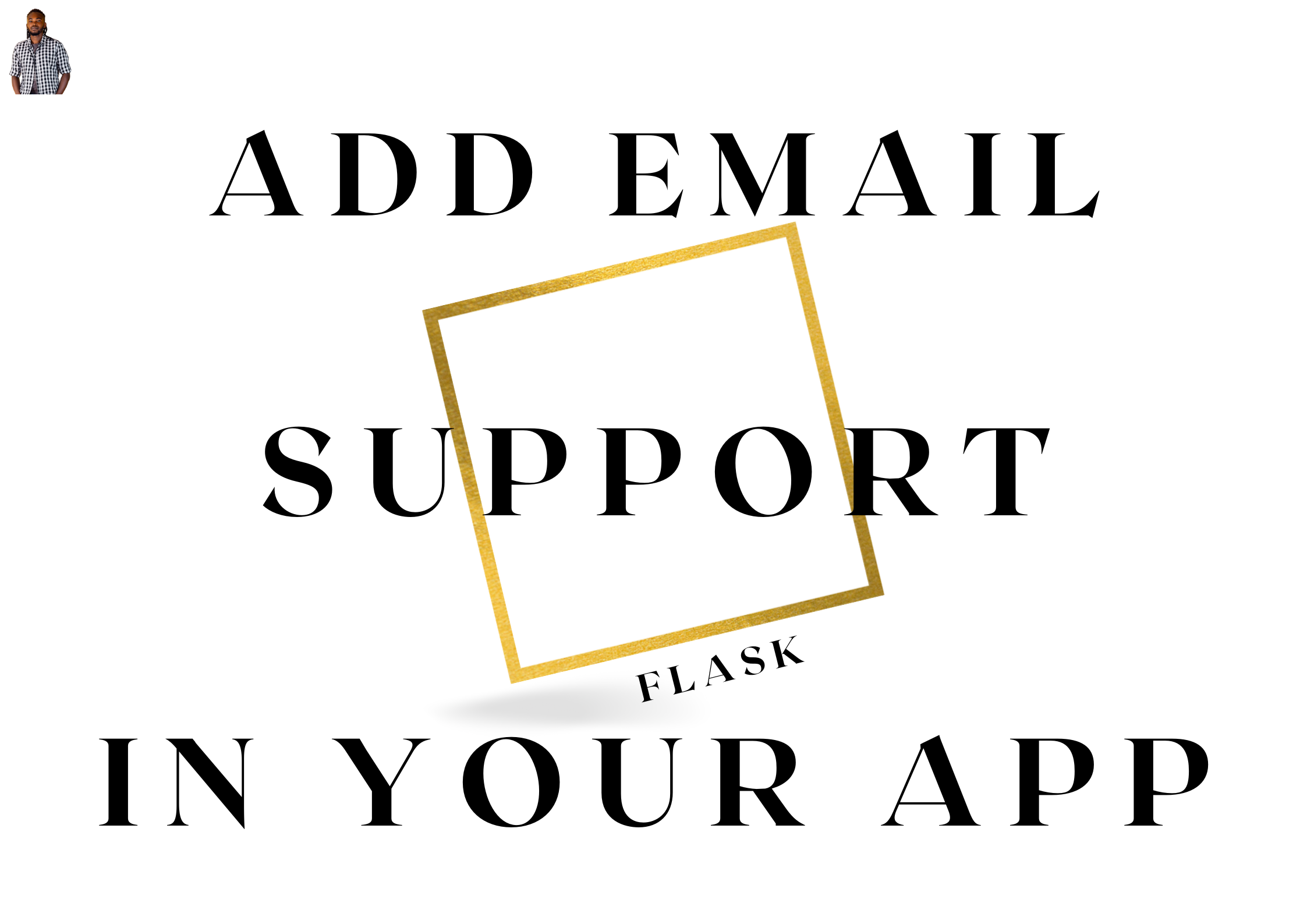
Email Support In Flask
Sending emails is a vital part of most web applications. There are many instances that may require the sending of emails in your application. For example, when a new user registers for an account, you may want to notify them of this action through the email they have registered with. Certain cronjobs may require the automatic sending of emails to an application's users at regular intervals. When it comes to user authentication, an application can also incorporate the ability to request a password reset.
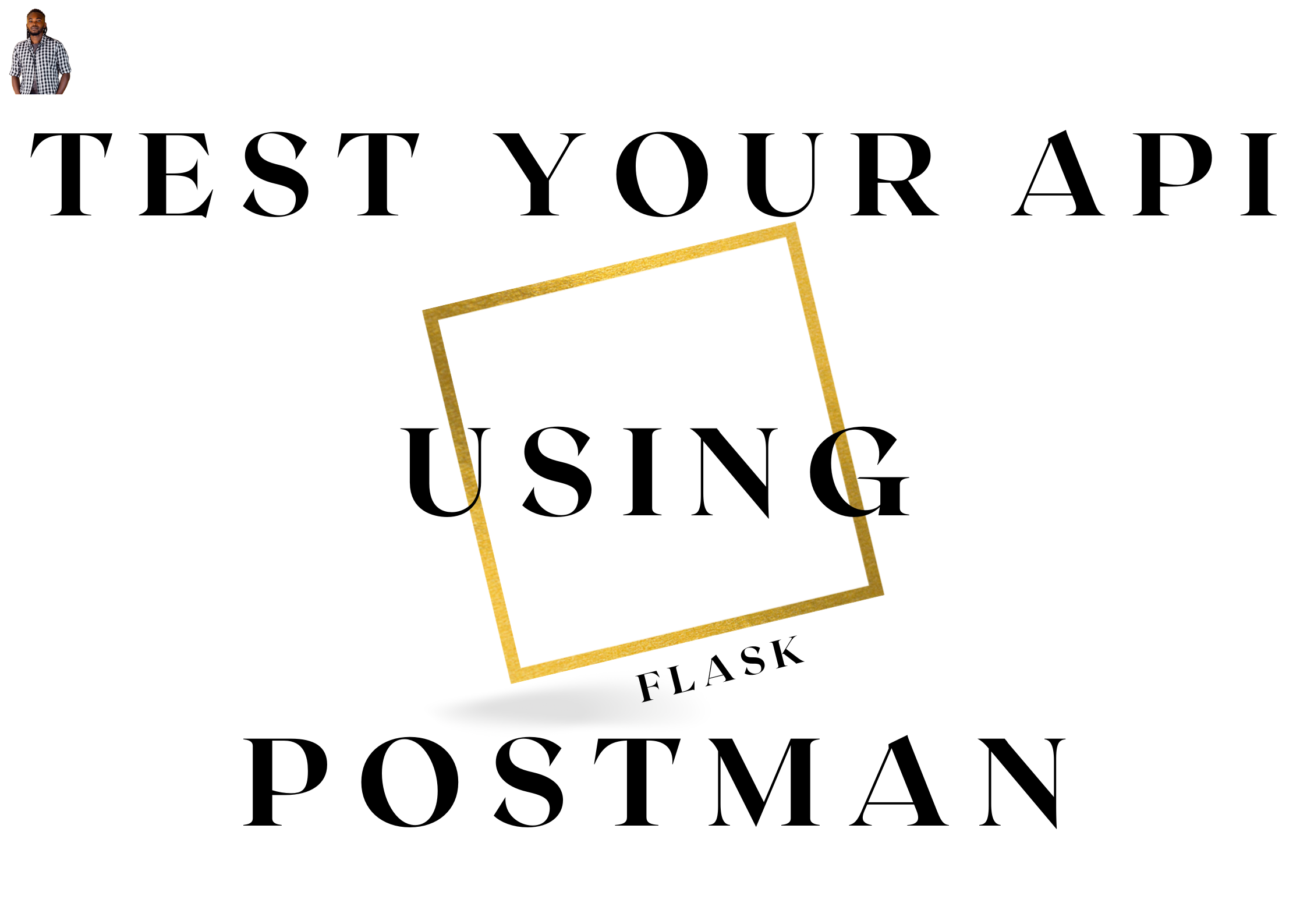
API Testing Using Postman
The HTTPie package is a fantastic command-line tool that can be used to test endpoints designed for testing, debugging, and generally interacting with APIs & HTTP servers. Another popular API-testing tool is Postman. It offers both a web and desktop client for creating, testing, sharing, and documenting APIs. While using Postman, for testing purposes, one doesn't need to write any HTTP client network code. Instead, we build test suites called collections and let Postman interact with the API.
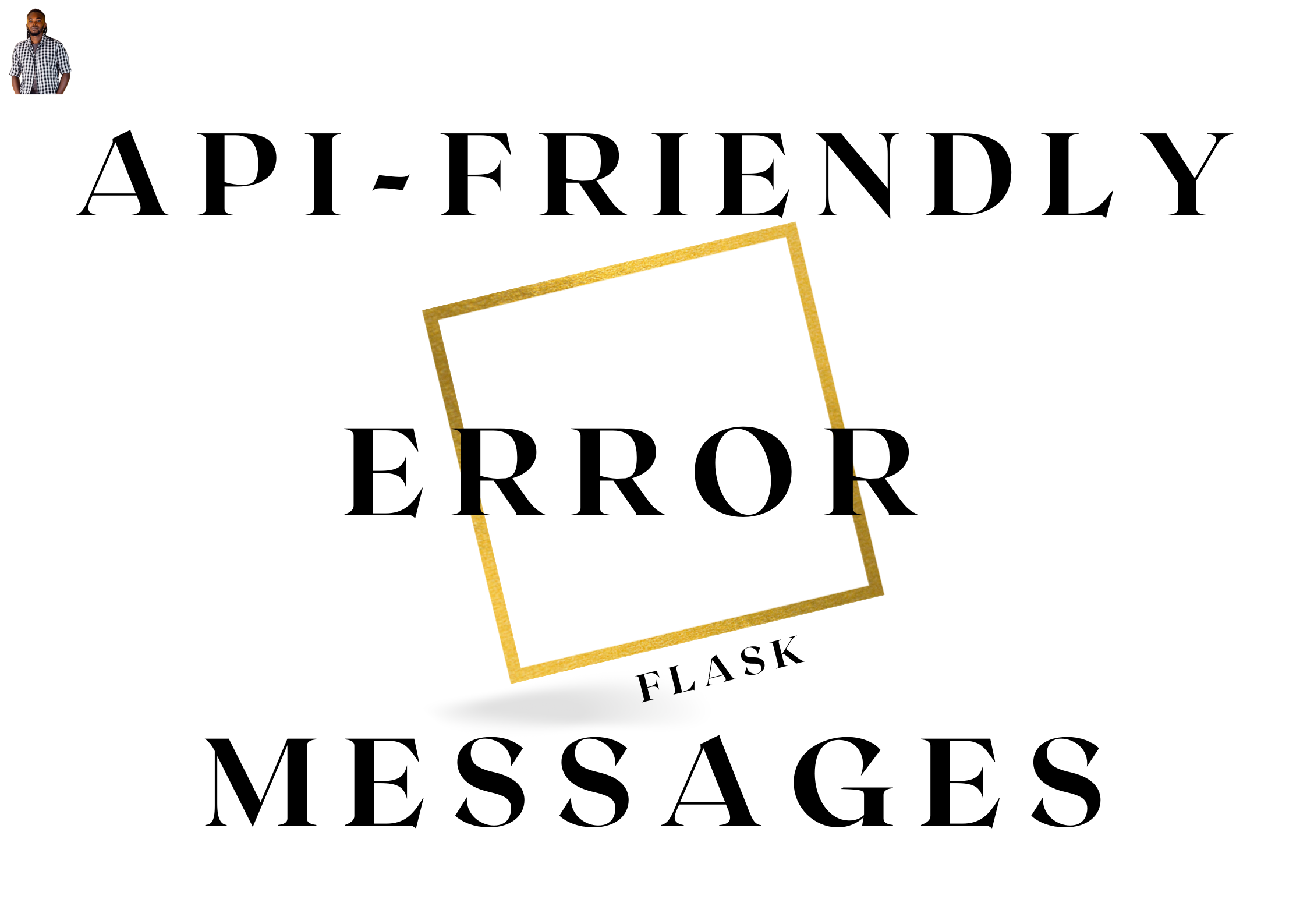
API-friendly Error Messages In Flask
We can go to extra lengths to provide custom error-handling mechanisms in an API, but there are some errors that would bypass these efforts and instead return HTML responses.
The HTTP protocol supports a mechanism by which the client and the server can agree on the best format for a response, called content negotiation. The client needs to send an Accept
header with the request, indicating the format preferences. The server then looks at the list and responds using the best format it supports from the list offered by the client.
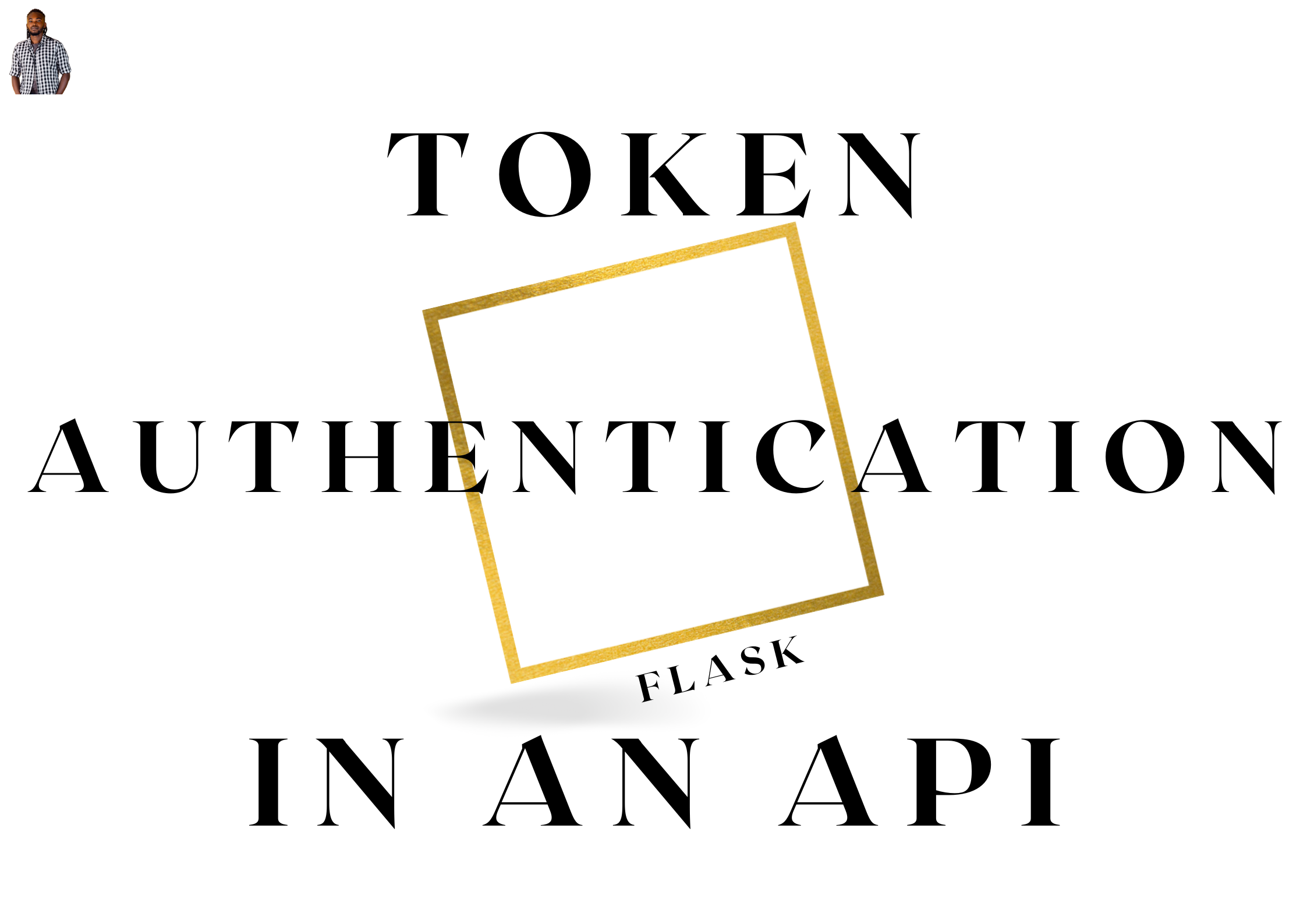
API Authentication In Flask
In a typical web application, there are certain web pages that require a user to login in order to access their content. Such pages require a client to identify, authenticate, and authorize a user in an effort to preserve their integrity. Traditionally, an anonymous user will be redirected to an HTML login page from where they will be verified.
It is also possible to achieve the same level of security when it comes to accessing an application's endpoints. Because there is no concept of HTML or login pages in an API, the server needs to return an appropriate response to the client to inform a user that they need to supply valid credentials.
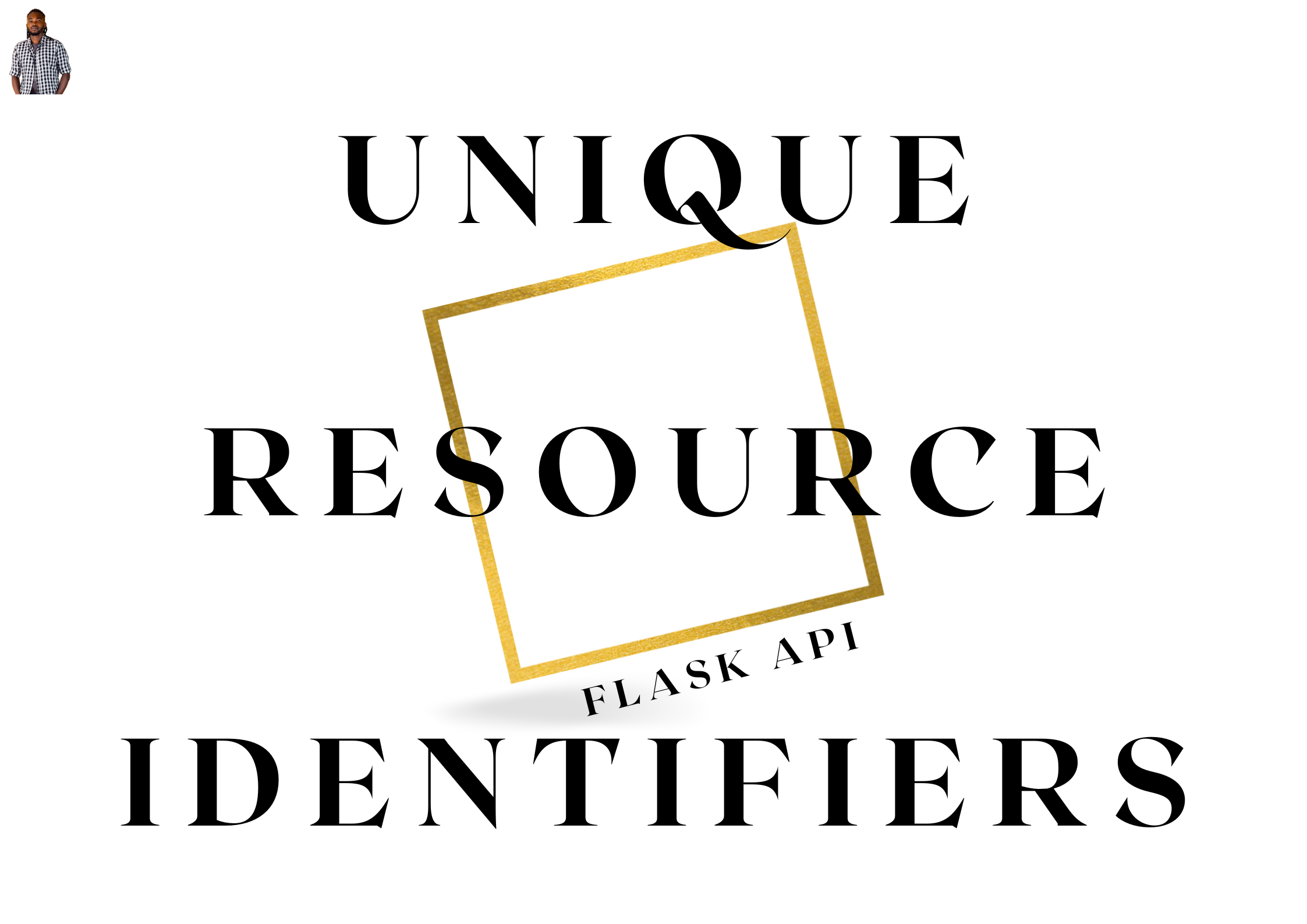
Unique Resource Identifiers In Flask API
A URI (Uniform Resource Identifier) is a string of characters that identifies a resource on the Internet. They are achieved by assigning a unique URL to each resource. For example, if a database has a user identified by the username "muthoni", her unique URL would be something such as /users/muthoni
where muthoni
is the identifier assigned to the user in the database. Such an endpoint can be reasonably implemented in an API.
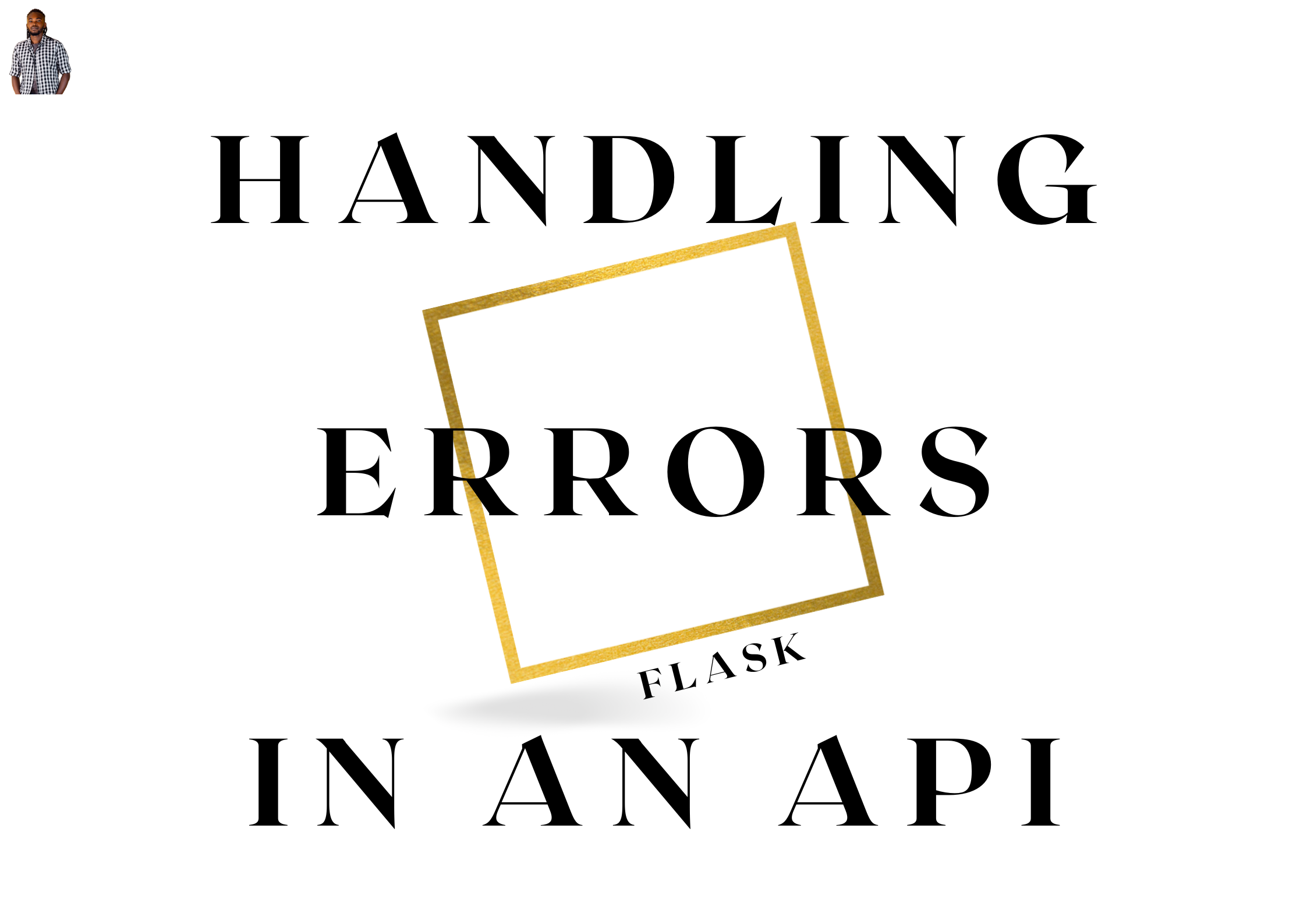
Handling Errors In Flask API
What happens when an error occurs in a Flask application? We would create custom HTML error pages such as 404.html
or 500.html
that display more user-friendly content. Flask would return these pages instead of a rather scary and oftentimes too revealing stack trace.
In the context of APIs, we would need a "machine-friendly" type of error, something that the client application would easily interpret.
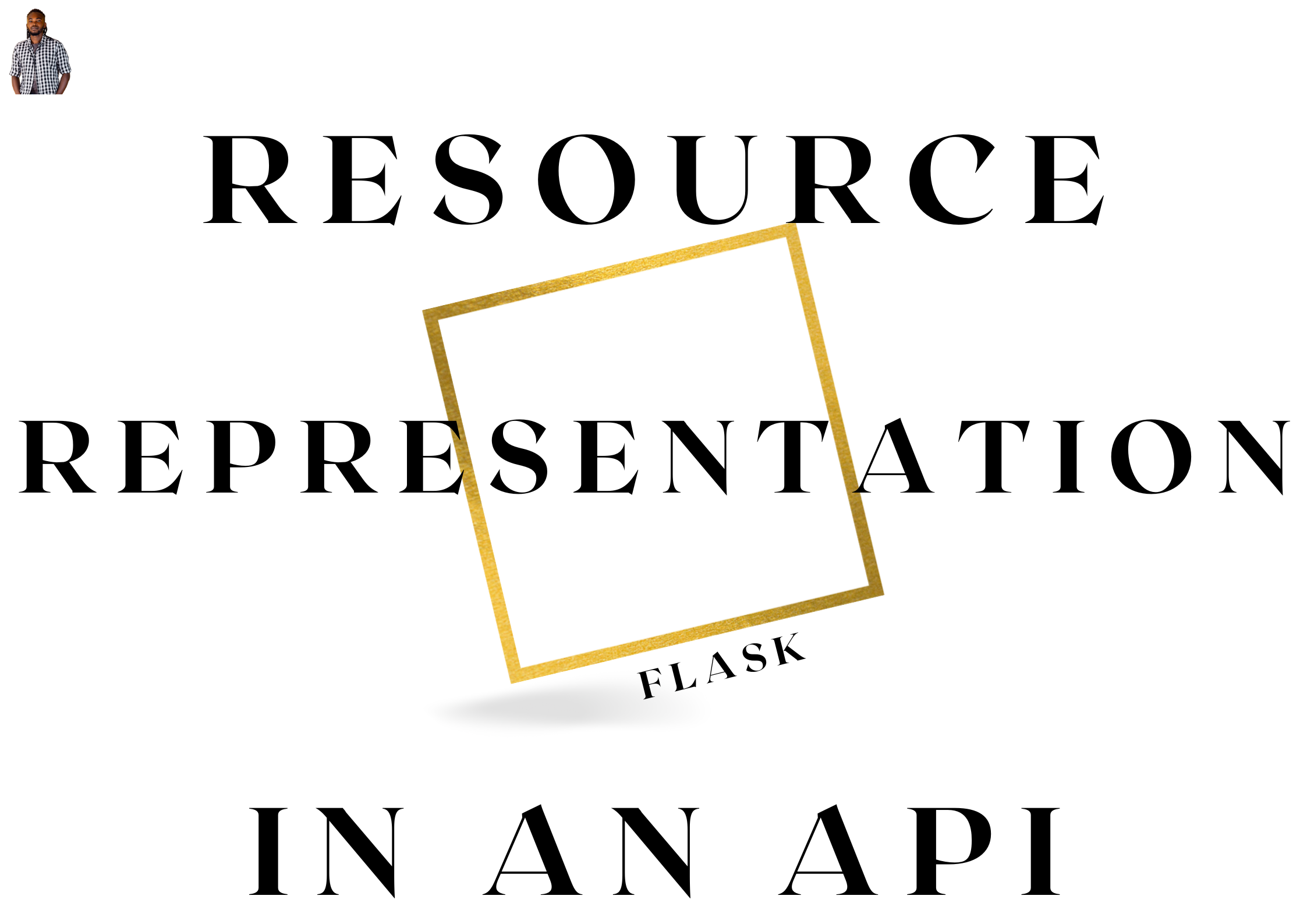
Representation Of Resources In Flask API
Before building any API, it is important to decide what the representation of its resources is going to be. Both the server and the client must agree on a format to represent a resource. Most modern APIs use the JavaScript Object Notation (JSON) format to build resource representations, though an API can support multiple representation formats when properly defined in their content negotiation options.
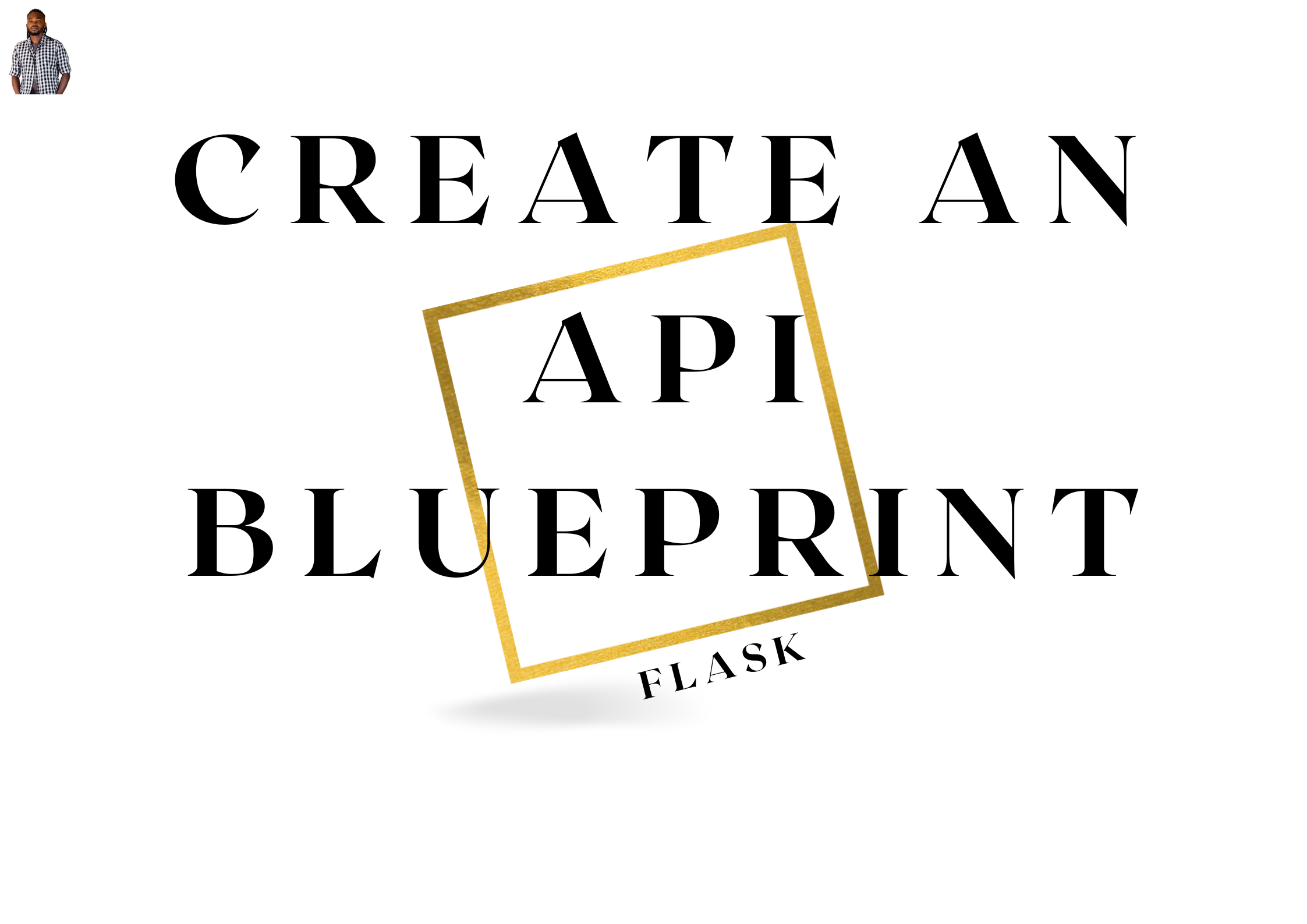
Implement An API Blueprint In Flask
A blueprint is a logical structure that represents a subset of the application. We can write a blueprint in a separate Python package that encapsulates the elements related to that specific feature of the application, in this case, an API. The essence of using blueprints in your Flask app is to allow for the adaptation of the application as it becomes larger or as the application's needs change.
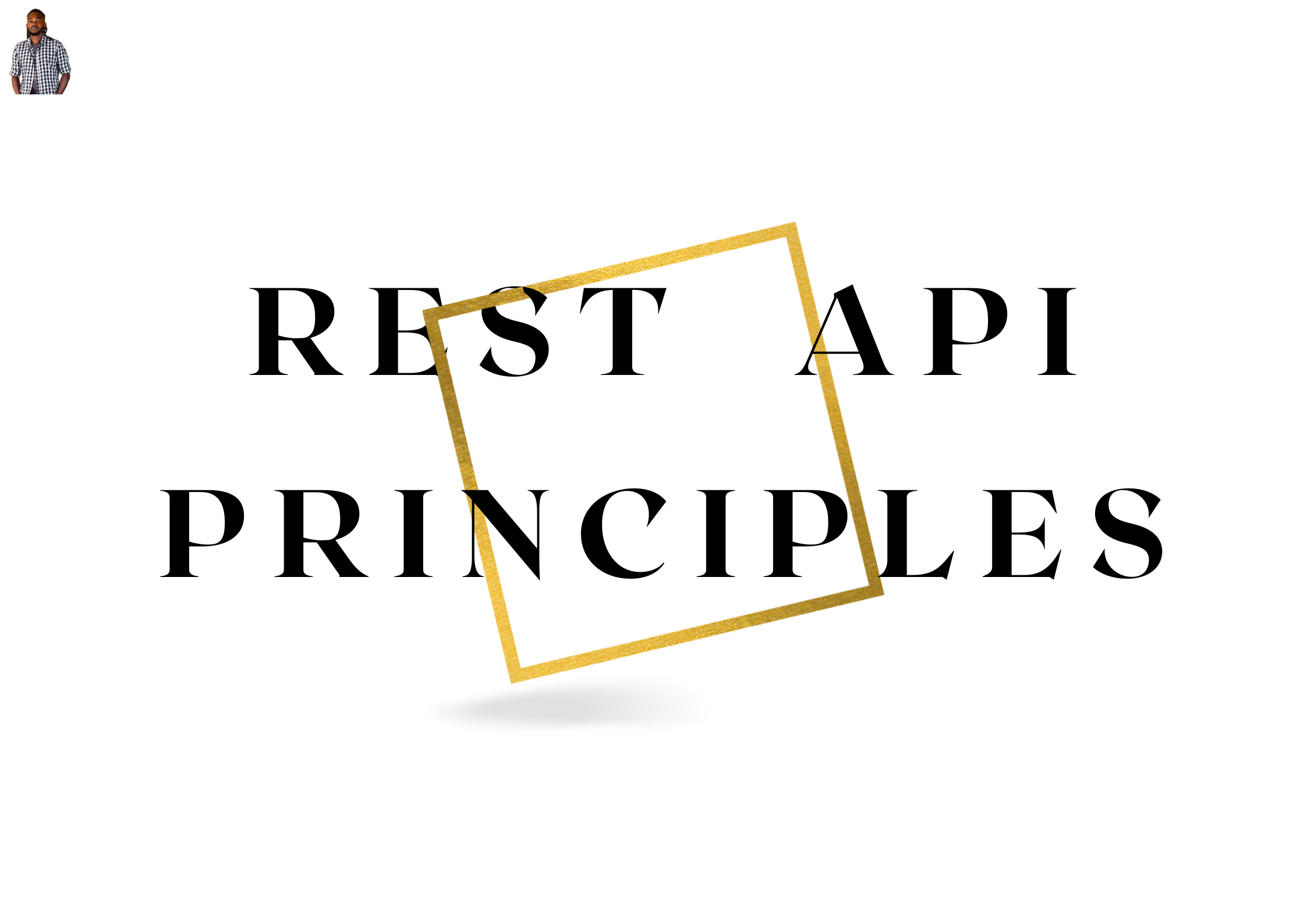
Application Programming Interfaces (APIs) In Flask
An Application Programming Interface is a low-level entry point into an application that a client can use to work with the application in a more direct way than through the traditional web browser workflow. Think of it as an intermediary layer that processes data between systems, through which a company can share its application's data and functionality with external third-party developers, partners, or even internal departments.
In this article, you will learn the basic principles that help guide the development of an API. There are several types of APIs. We will examine the most popular API among them all, the REST API.
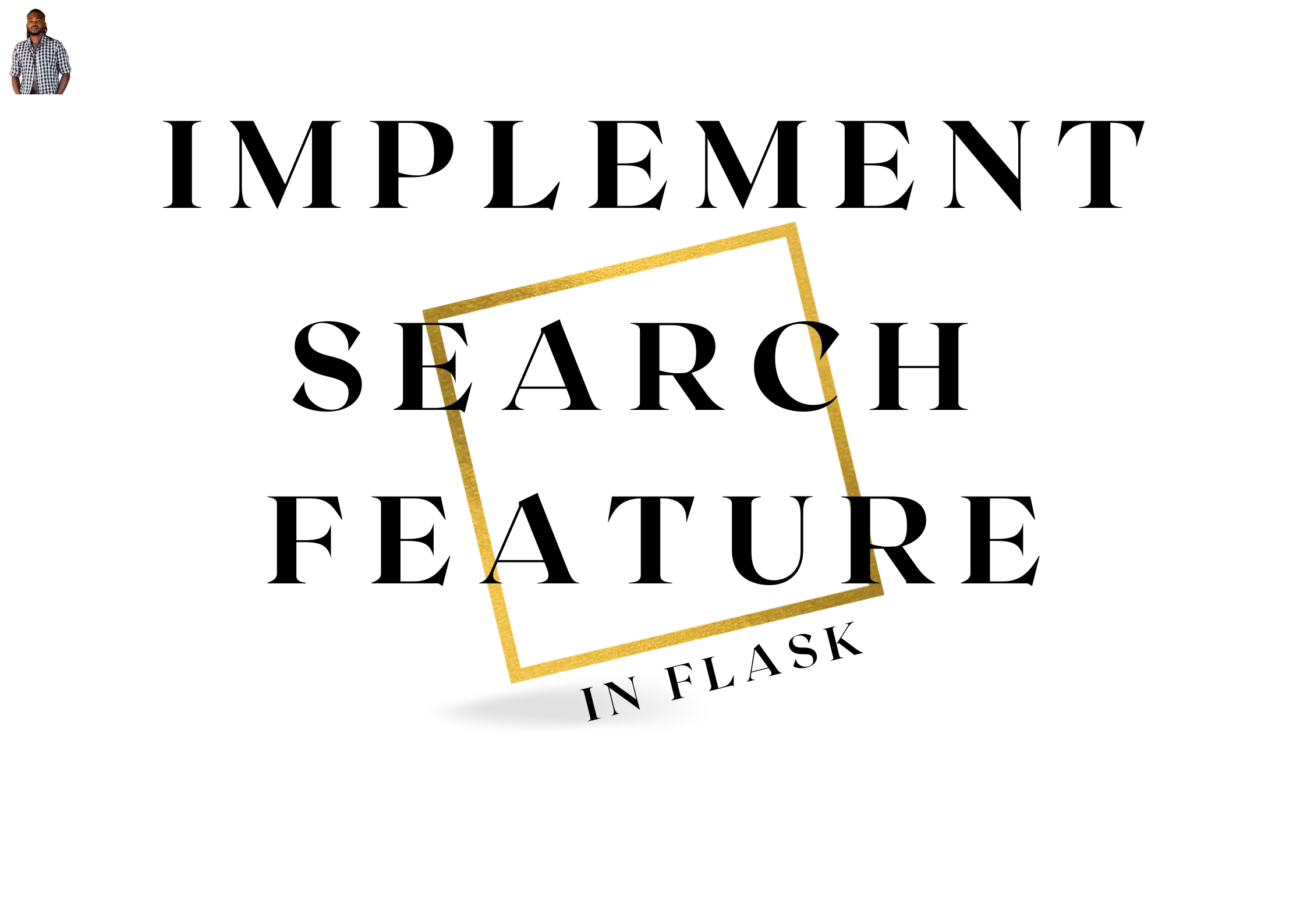
Implement Search Feature In Your Flask App Using Elasticsearch
The search feature can dramatically improve a user's experience while interacting with an application. A simple form can be added to a web page or the navigation bar to allow a user to quickly retrieve desired results. In this article, you will learn how to implement a very generic approach to building a search functionality such that you can easily switch from one search engine to another.
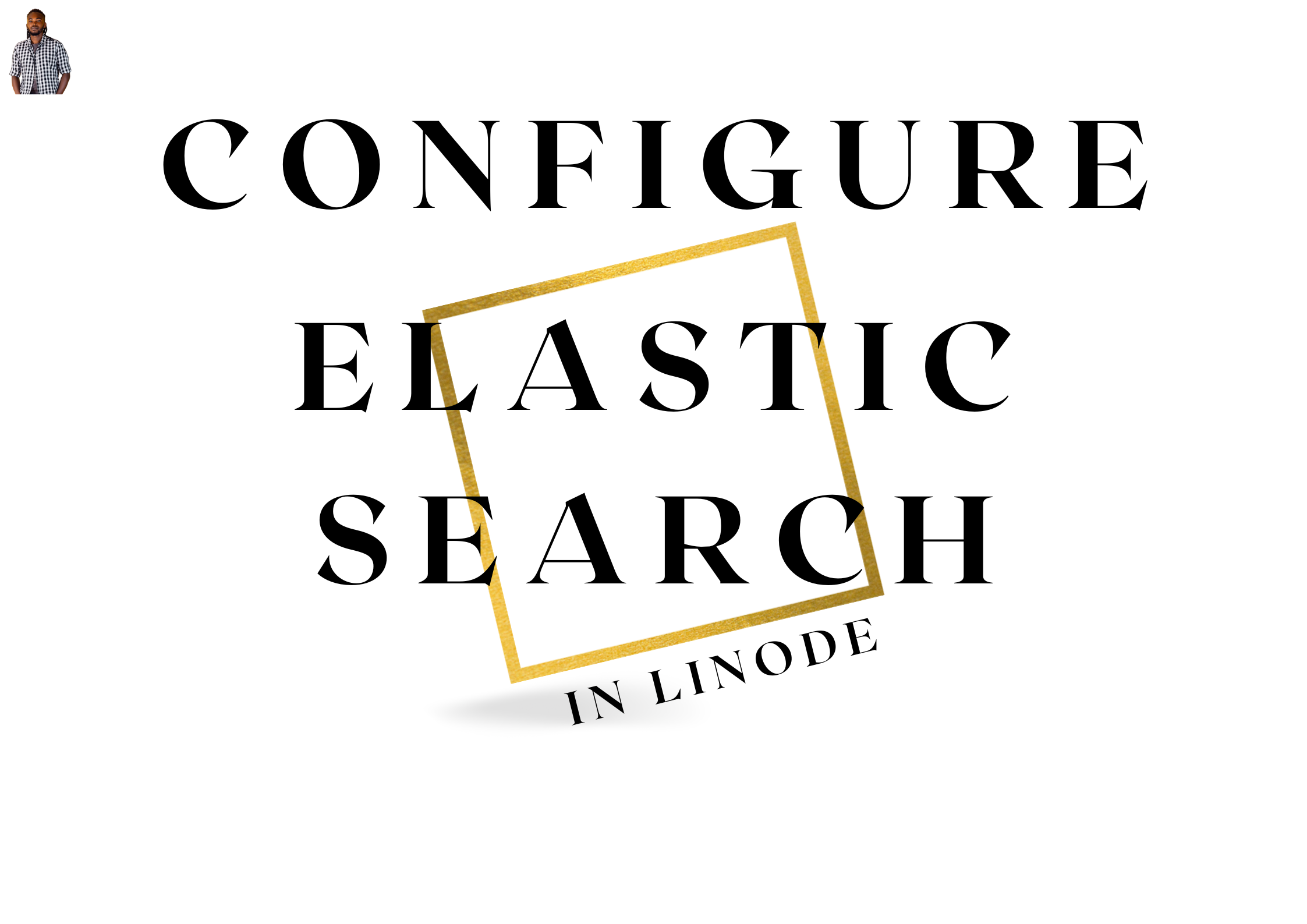
Install And Configure Elasticsearch In Your Linux Server
If you are personally hosting your Python-powered app, you will have to contend with configuring Elasticsearch manually to ensure it works in production. As with any other deployment on a Linux server, the process of configuring yet another service can be intimidating. In this article, you will learn how to get your Elasticsearch service to work on Linode.
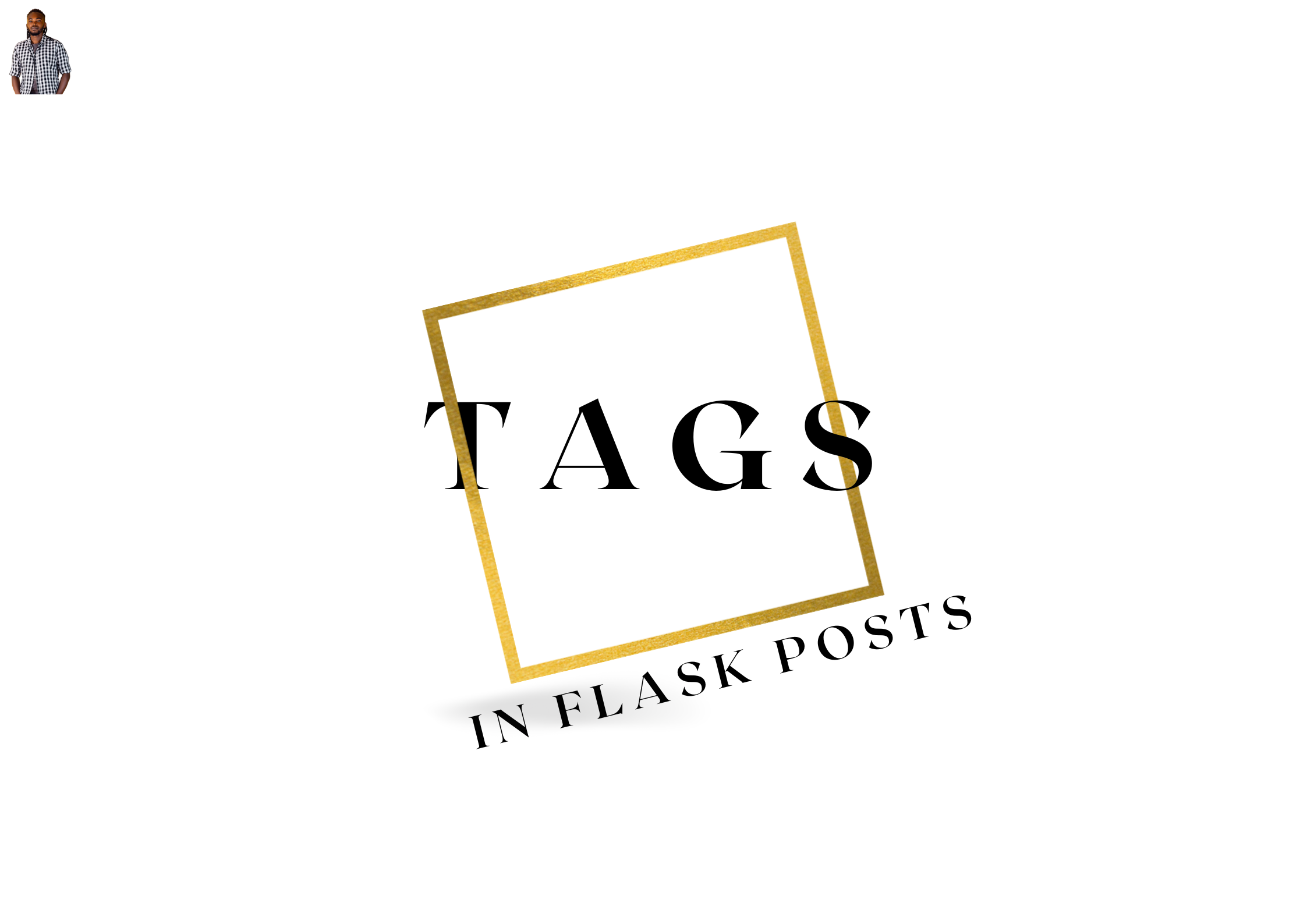
Add Tags To Your Posts
Tags are a simple classification system that can be used in a blog post. A tag can be applied to multiple blog posts and, similarly, multiple blog posts can be related to one another outside their category. For example, a blog may have a tag called "Flask". People interested in reading posts related to "Flask" only can click on this link and they will find relevant posts.
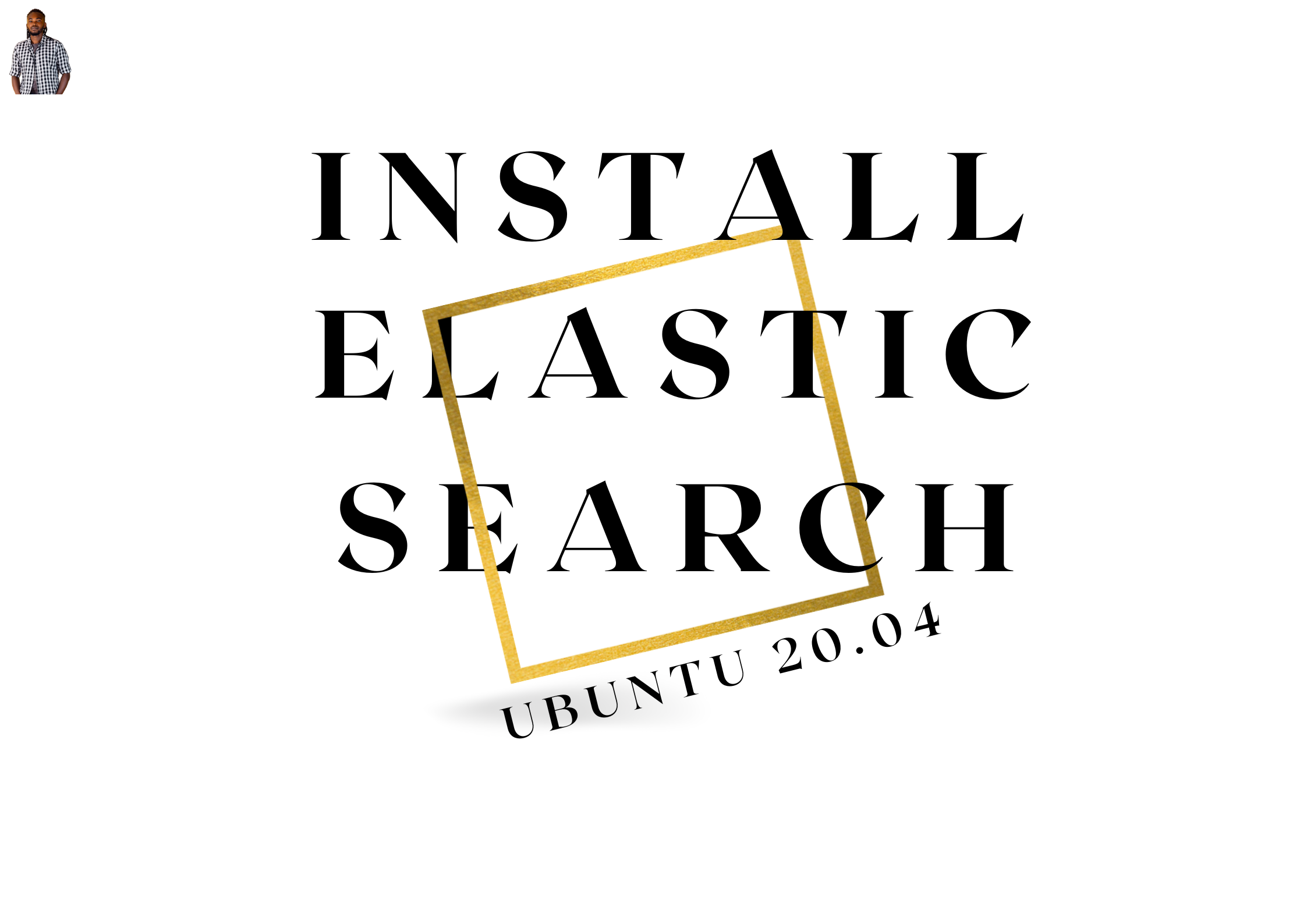
Install Elasticsearch In Ubuntu 20.04 Localhost
If you are looking at utilizing Elasticsearch, the free and open-source search and analytics tool, you have to familiarize yourself with the technical setup details. In this article, you will learn how to download, install and start the Elasticsearch service. You will also briefly learn how to use sample JSON data to ingest and index search queries in your Flask app.
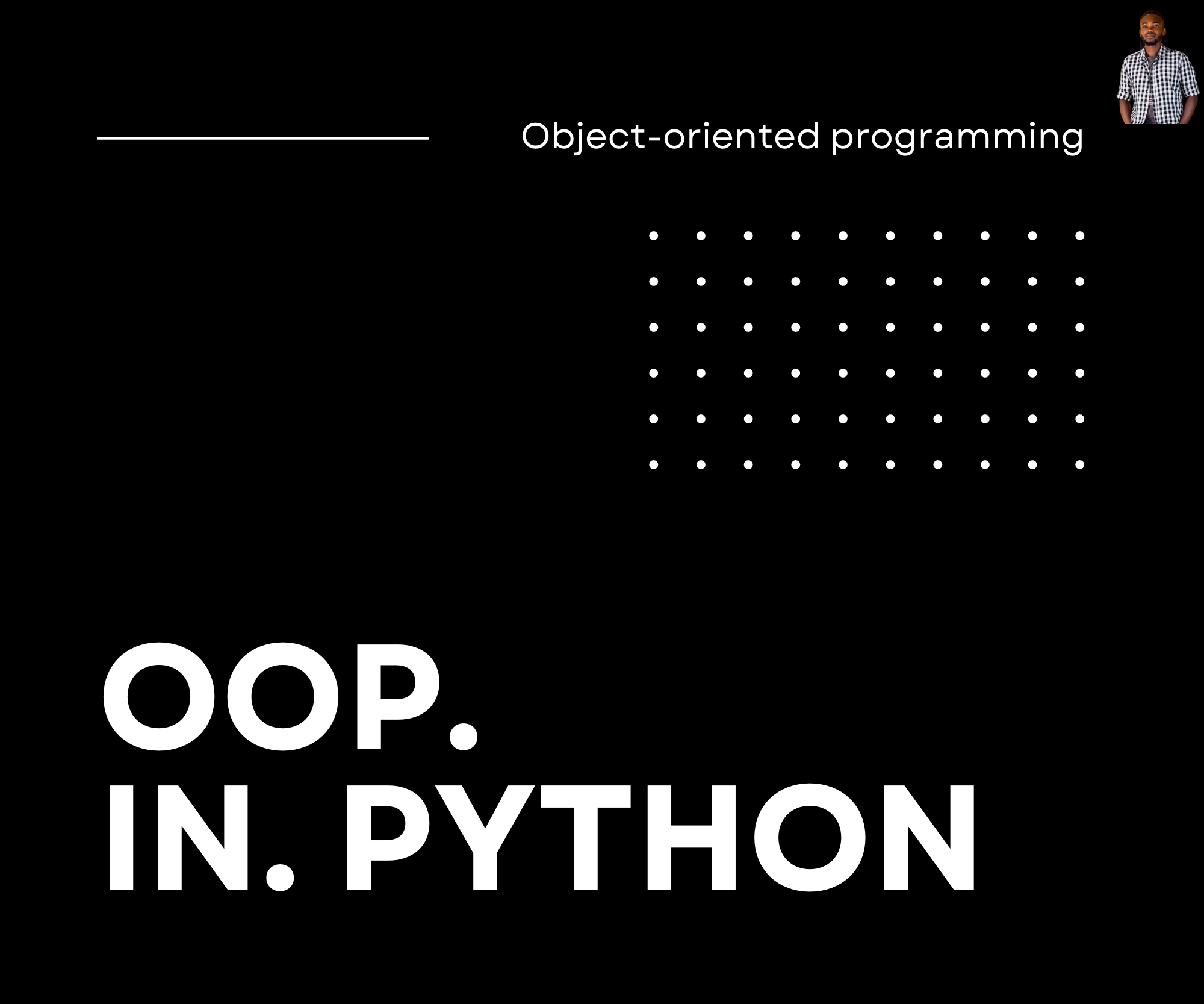
Object-oriented Programming In Python
Object-oriented programming, as the name suggests, is a method where related properties and behaviors are bundled into individual objects. These objects are instances of a class
that has generic attributes and methods used to define something. Think of a car, it has wheels and can move. The car is generic and can be used to define a Bentley or a Benz. In this article, you will learn how to create and use objects in Python.
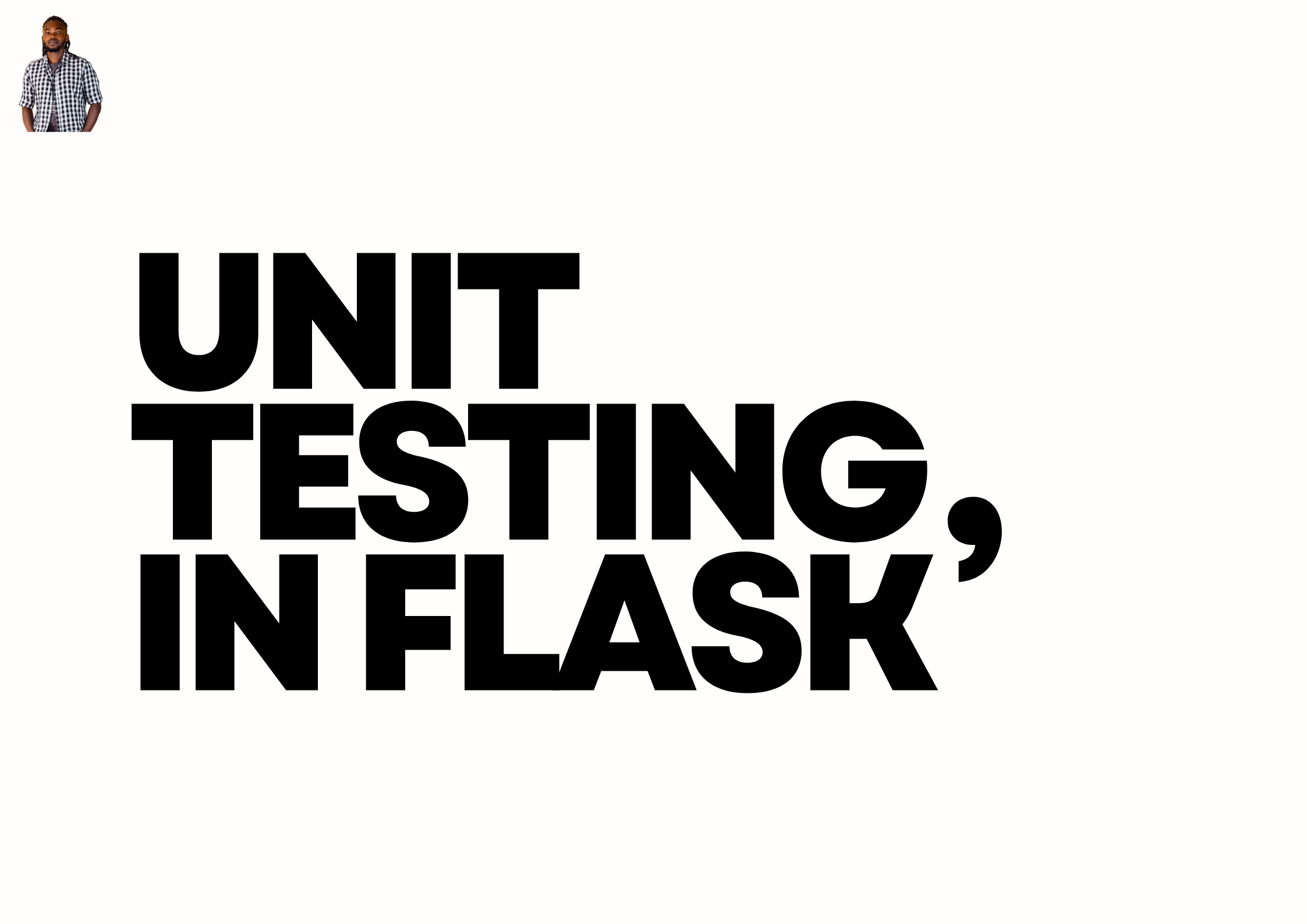
Unit Testing in Flask
Testing is so fundamental in good software development that there is even a process based on testing, called Test-driven development (TDD). TDD allows for test cases to be specified and validated prior to building an application. A TDD approach to software development is meant to encourage simple designs and inspire confidence.
A software developer is encouraged to begin by writing tests, which fail at the start for obvious and expected reasons. An effort is then made to write the simplest code that passes the new test. This change should see that the test case passes. Depending on the size of an application, the developer will need to refactor the code as much as needed using each test to ensure that the functionality is preserved.
The cycle is repeated for each new piece of functionality. Fundamentally, tests should be small and incremental, and commits made often. This allows the developer to undo or revert the failed tests than debug excessively.
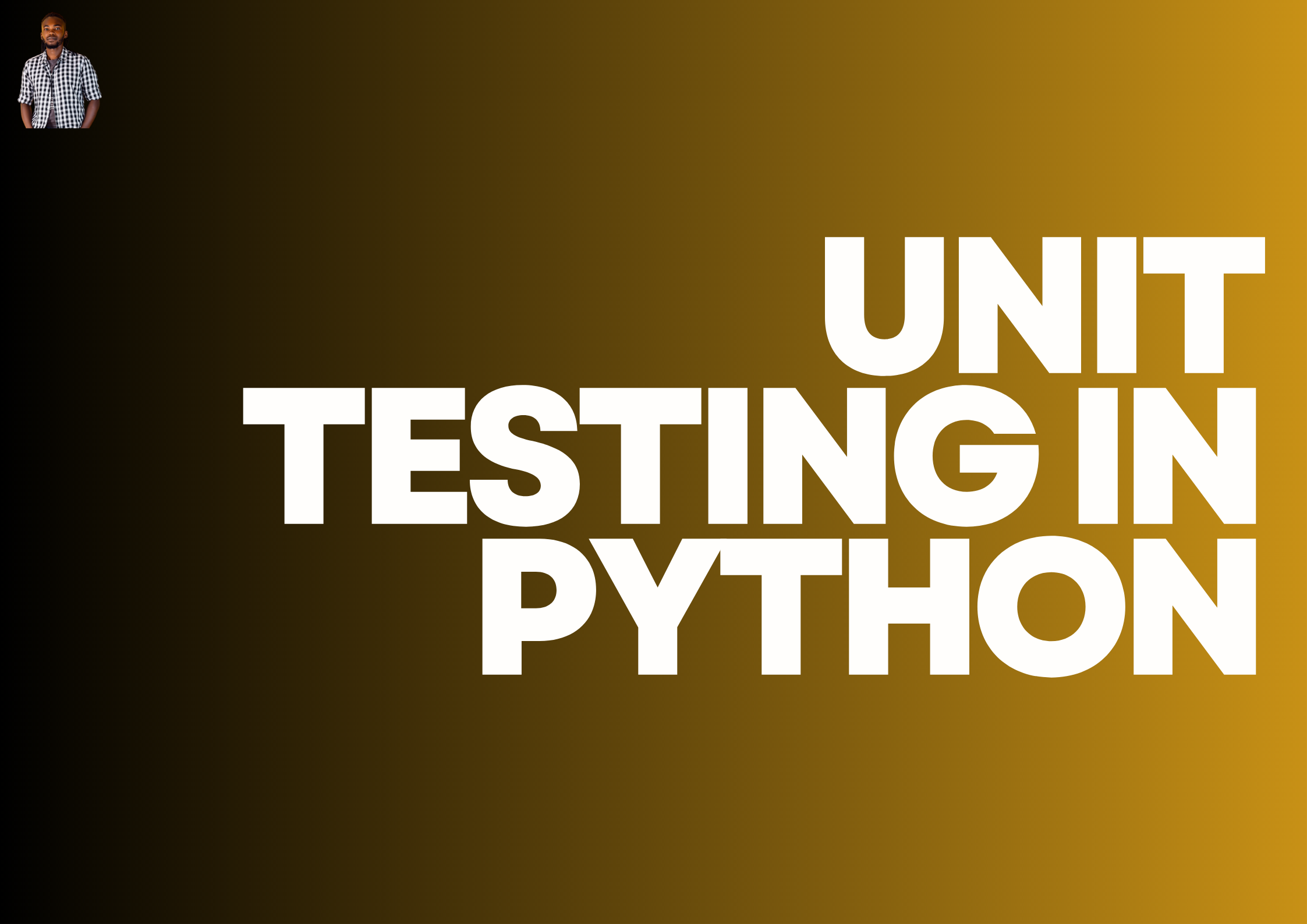
Unit Testing in Python
Unit testing is a development approach that looks at the smallest pieces of code, called units, in a software application that are tested for correct operations. When a unit is tested, you can verify that the code tested works as intended. In this article, you will learn how to implement unit testing on a Python script.
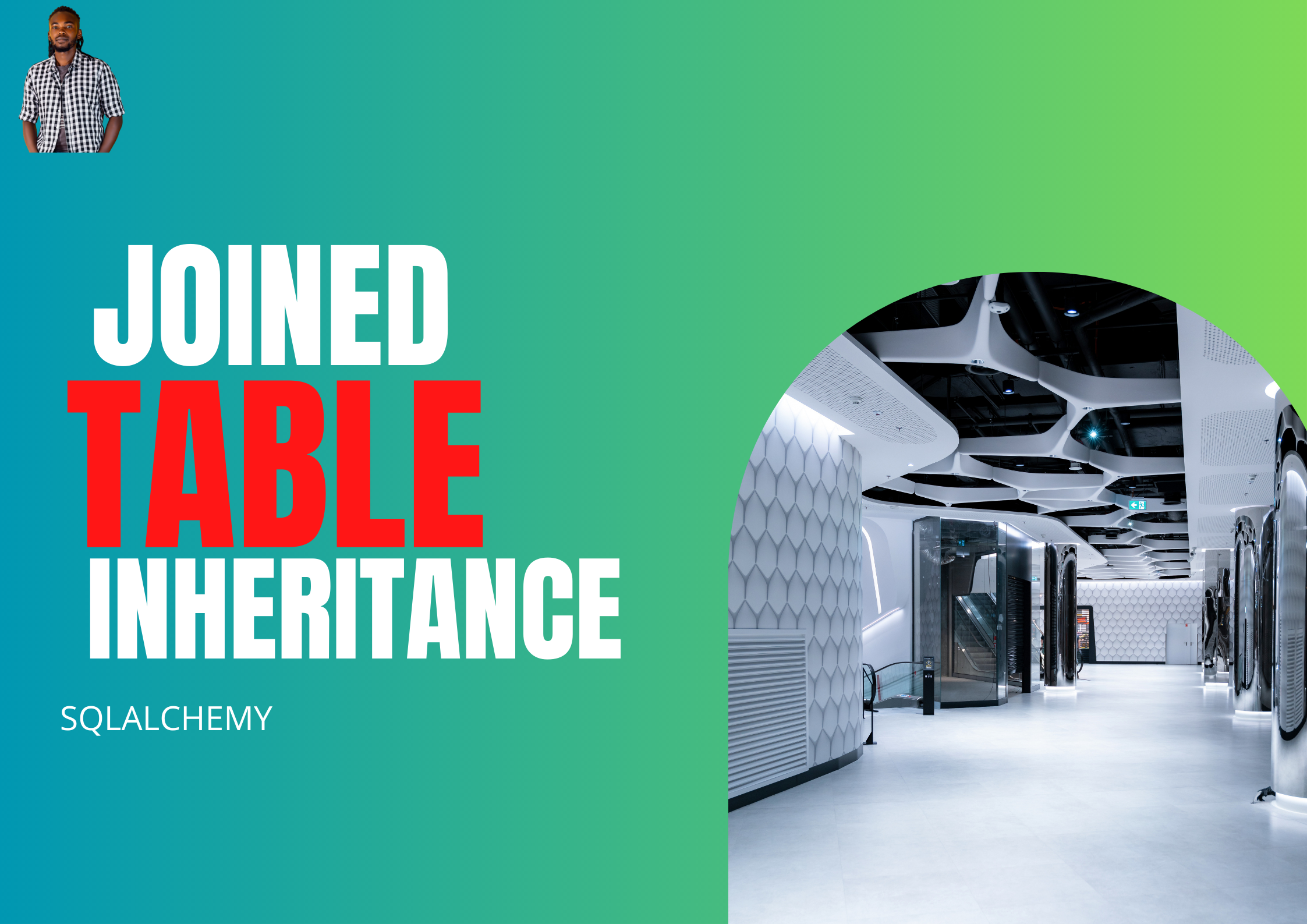
Joined Table Inheritance In SQLAlchemy
A properly designed database allows us to organize our data in the most efficient manner. We will also have the luxury to access up-to-date and well-curated data. Because a solid design is essential to achieving our goals when working with a database, it is essential that we invest the time to learn good design principles. Designing a database schema is a task that’s easier said than done—but by observing a few tips, principles, and best practices, we’ll be much better positioned for success. This article reviews the concept of Joined Table Inheritance from SQLAlchemy.
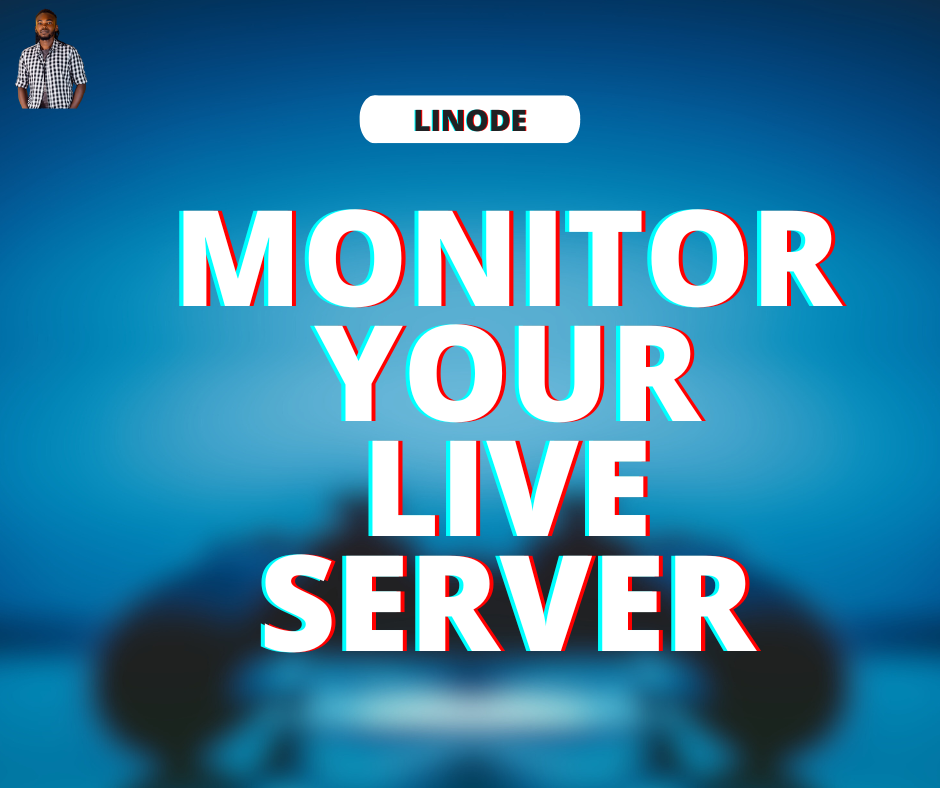
Monitor Your Live Server On Linode
Once your application is live in your virtual machine, the only way to know that your Linux-server-based applications and services are running well is to measure what’s going on in the server and its connectivity status. A handful of Linux monitoring tools are available to help reassure us that things are working right and that anomalies are quickly recognized. Mastery of these dedicated tools, most of which are already built into the operating system, places us well on the trajectory of Linux system administrator expertise.
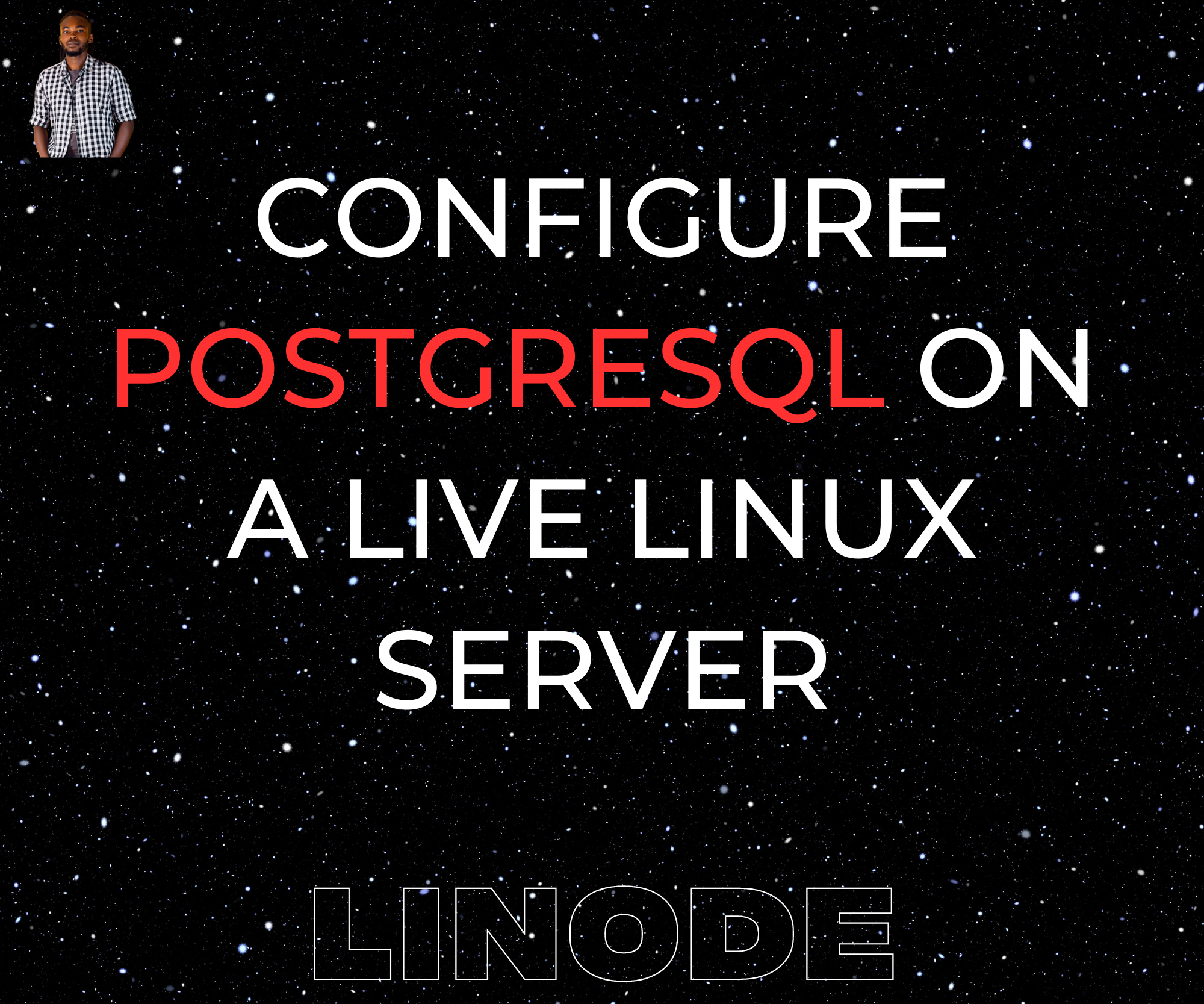
Configure PostgreSQL In A Live Linux Server
PostgreSQL is a feature-rich application, offering advanced features such as materialized views, triggers, and stored procedures. It can handle a very high workload, including data warehouses or highly-scaled web applications, and is noted for its stability.
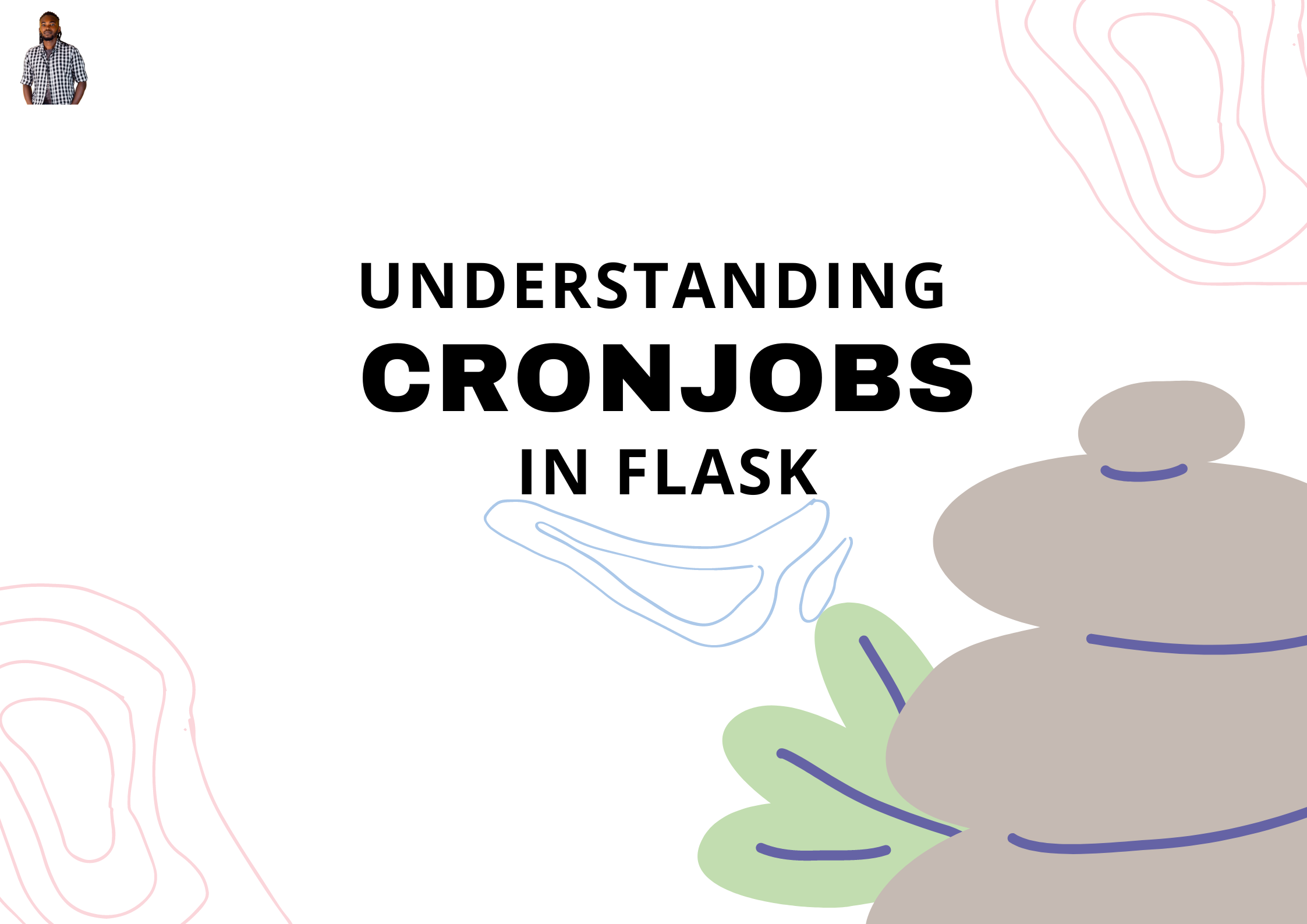
Understanding Cronjobs in Flask
It is common for web applications to run background tasks. The applications could need to clear unnecessary files or cache, import third-party data, or even periodically send out email newsletters. Thankfully, all Unix-based have a scheduling utility that makes it possible to automate certain tasks.
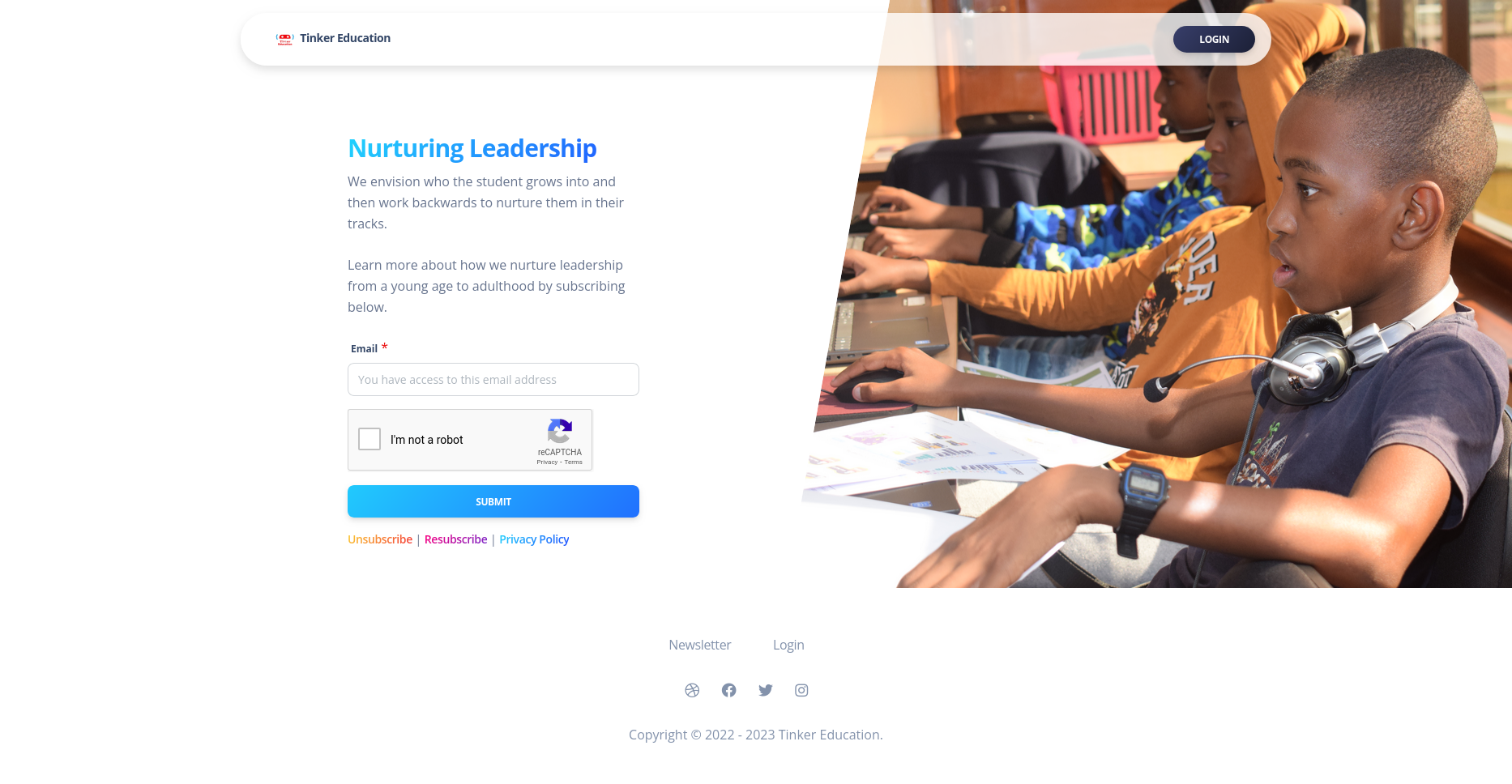
Tinker Education Elearning App
A newsletter application plays an important role when launching a new product. As a business, you'd want to collect user interest in your new product or service prior to the launch for the purposes of decision-making.
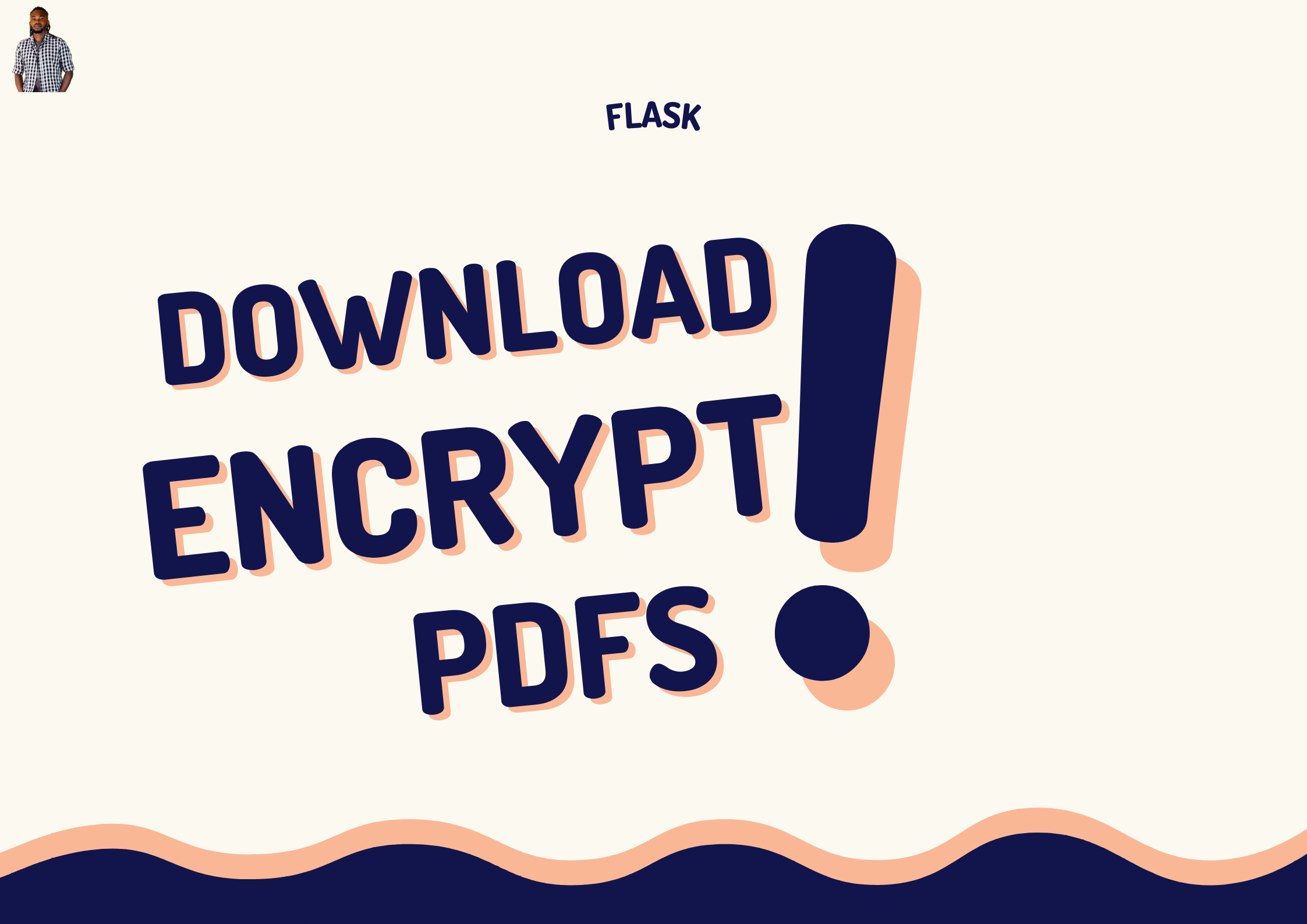
Download and Encrypt PDFs in Flask
PDF documents are not editable by nature. They are the sole read-only file format that can’t be altered without leaving a footprint. This allows the effective tracking of changes in respective documents. Encryption can be enabled in the document to protect it wherever it goes, independent of storage or transport. As complex as this entire document creation and encryption process seems to be, Flask allows for the integration of this feature in an application.
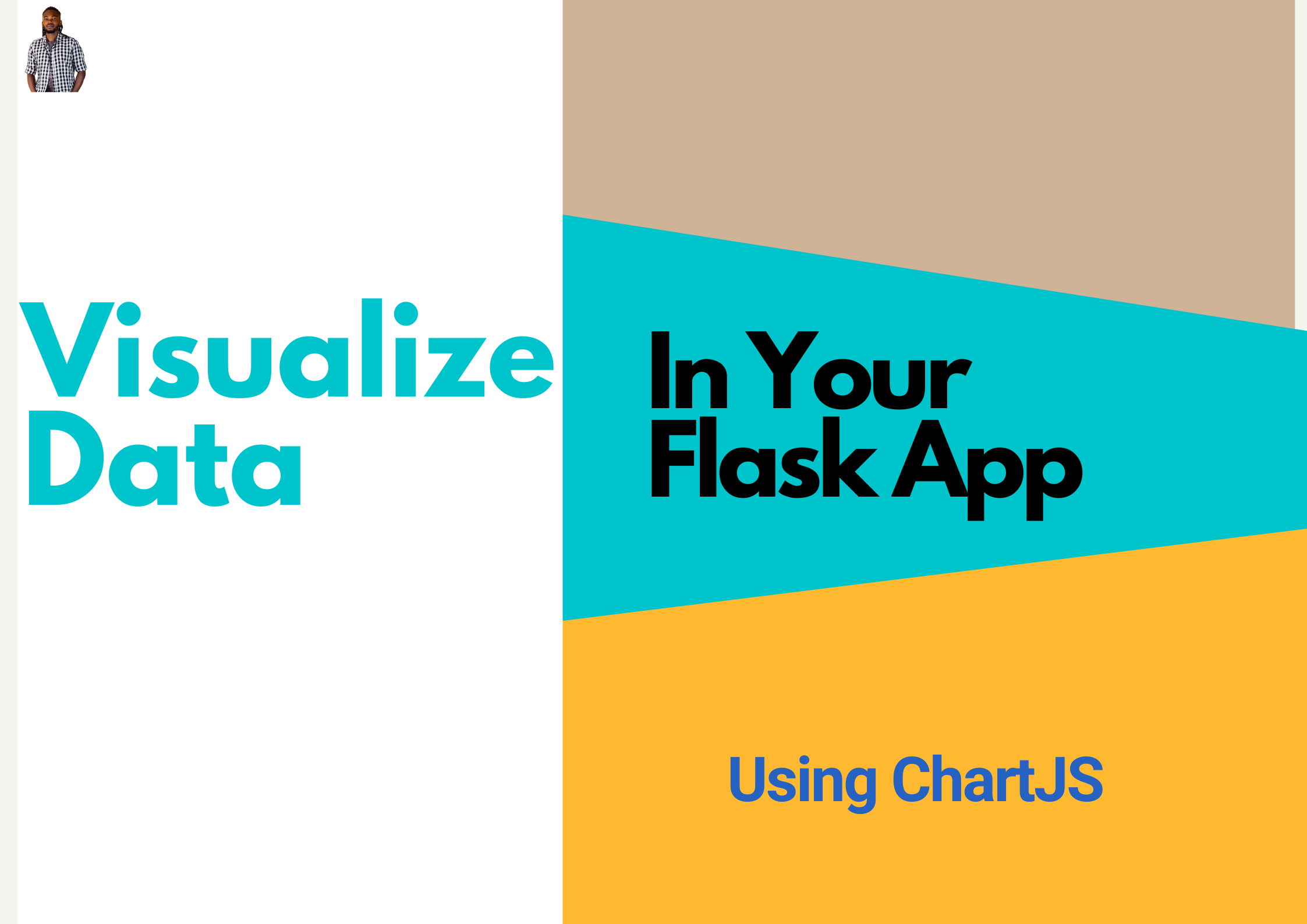
Visualize Data In Your Flask App Using ChartJS
Data visualization is the graphic representation of information. This could be in the form of a chart, diagram, map, or picture. In the world of Big Data, data visualization tools and technologies are essential for analyzing large datasets and making data-driven decisions.
ChartJs is a free and open-source JavaScript library used to make HTML-based charts. It is powerful enough to reproduce very responsive charts and plots. You can use it to plot static and constantly changing data.
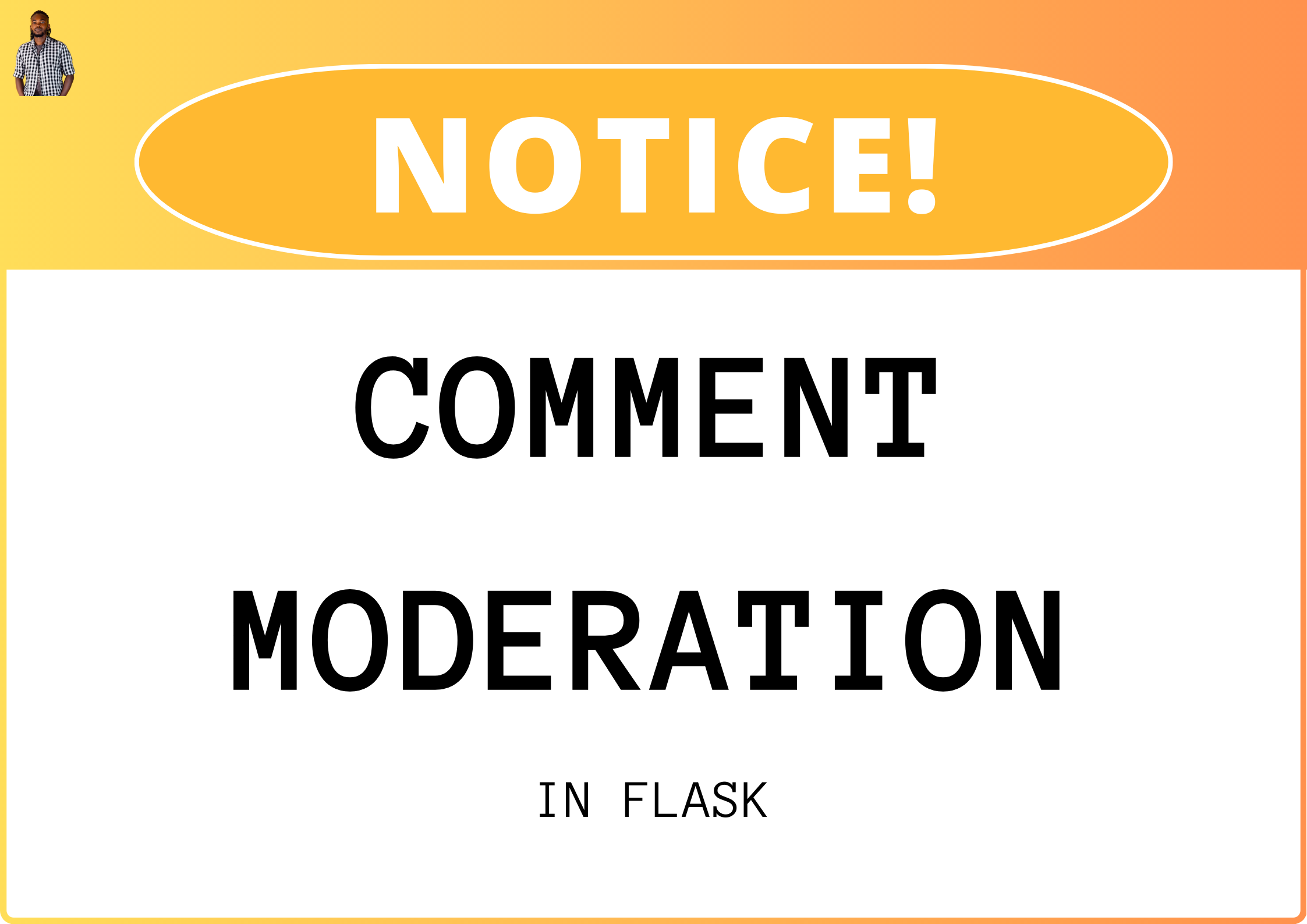
Comment Moderation In Flask
As an administrator of an online application, have you been worried about the quality of comments that appear on your site? Comment moderation is an attempt to promote healthy conversations on your platform.
Typically, a user may register and jump into the comments section to experience the application's community. To ensure that warm engagements are maintained throughout, moderators will have first access to all user comments before allowing them into the community.
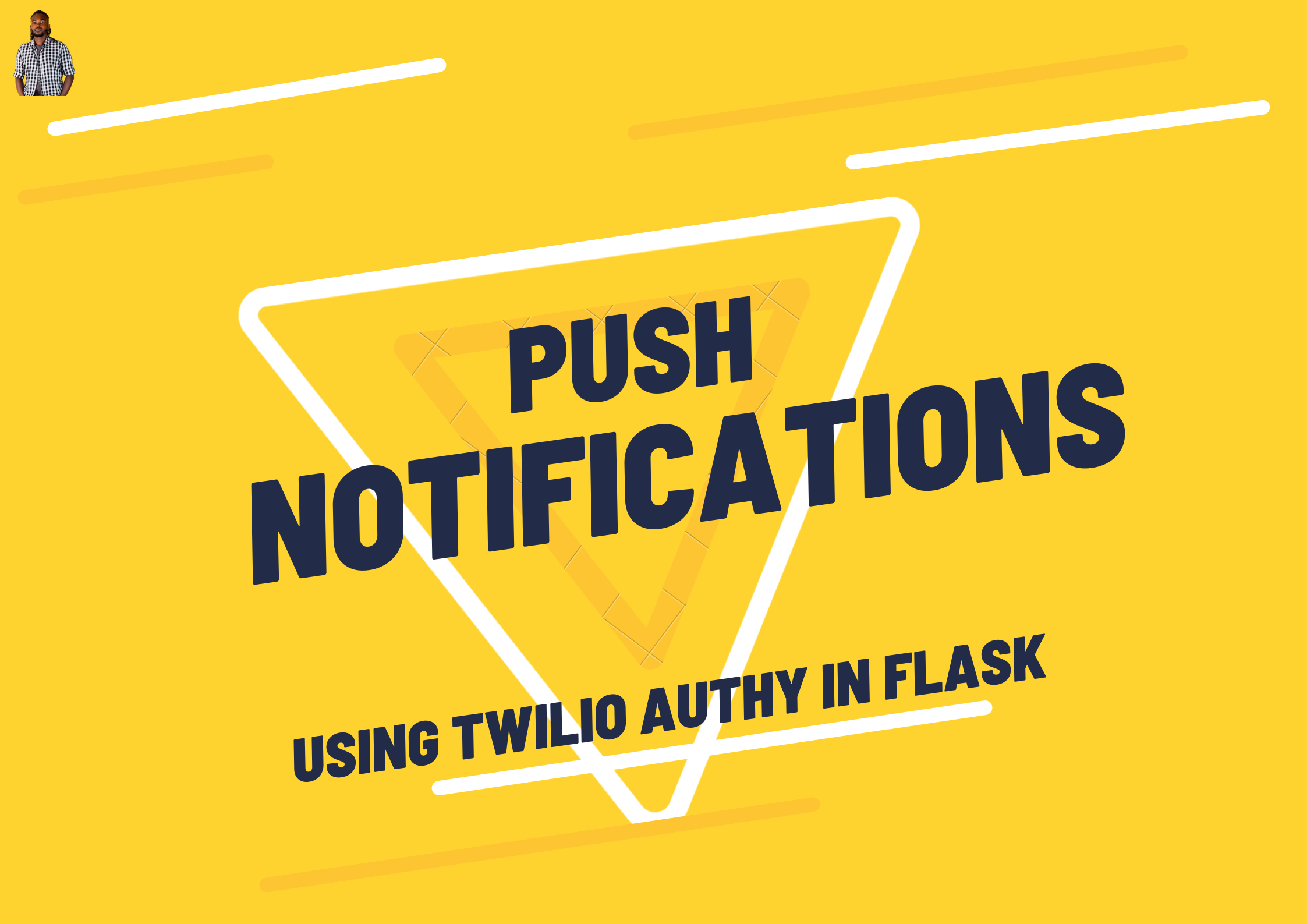
Push Notifications Using Twilio Authy API In Flask
Push notifications are messages that pop up on a user’s mobile phone or desktop device via their chosen web browser. These little banners slide into view — whether or not your app or website is open.
Push notification can also be used to authenticate a user silently without requiring their active participation in the authentication process as is the case with token-based methods. All a user needs to do is click a button to either approve or deny access to an account.